利用JSzip进行多类型文件下载(pdf、图片类型)
封装下载zip逻辑代码,可多处重复使用
import JSzip from 'JSzip'
import { base64ToBlob } from './base64ToBlob'
import type { DownloadList } from '@/views/audit/element/types'
/**
* 下载zip文件
* @param fileName 文件名(不需要加后缀){string}
* @param downloadList 压缩包内文件的列表 {DownloadList[]}
*/
export function downloadZip(fileName: string, downloadList: DownloadList[]) {
const zip = new JSzip()
const fileNames: string[] = []
downloadList.forEach((item) => {
const filename = item.fileName
const blob = base64ToBlob(item.url, item.suffix)
fileNames.push(filename)
// 判断是否有重复数据
const find = (fileNames: string[]) => new Set(fileNames).size !== fileNames.length
if (find(fileNames)) {
// 有重复的在创建一个文件夹并拼接时间戳
const folder = zip.folder(`${item.fileName.split('.')[0]}_${Date.now()}`)
folder?.file(filename, blob, { binary: true })
}
else {
zip.file(filename, blob, { binary: true })
}
})
// 在添加完所有文件后生成 ZIP 文件
zip.generateAsync({ type: 'blob' })
.then((content: BlobPart) => {
const zipFile = new Blob([content])
const link = document.createElement('a')
link.href = URL.createObjectURL(zipFile)
link.download = `${fileName}.zip`
link.click()
})
.catch((error: Error) => {
ElMessage.error(`创建 ZIP 文件时出错 ${error}`)
})
}
封装base64转成blob格式逻辑代码
/**
*
* @param str base64字符
* @param suffix 后缀格式(可选)默认为pdf格式
* @returns new Blob
*/
export function base64ToBlob(str: string, suffix?: string) {
str = str.replace(/[\n\r]/g, '')
const raw = window.atob(str)
const rawLength = raw.length
const uInt8Array = new Uint8Array(rawLength)
for (let i = 0; i < rawLength; ++i)
uInt8Array[i] = raw.charCodeAt(i)
return new Blob([uInt8Array], { type: suffix === '.pdf' || !suffix ? 'application/pdf' : 'application/octet-stream' })
}
具体使用
const actionConfig = ref([
{
label: '下载',
type: 'download',
click: (row: any) => {
if (row.fileIds) {
// 根据id获取到需要下载的文件路径
downloadList.value = []
const file = row.fileIds.map(async (item: any) => {
return getListById(item).then((res) => {
downloadList.value.push({
fileName: res.data[0].name,
url: res.data[0].bytes,
suffix: res.data[0].type,
})
})
})
// 等待上边代码执行完后再执行
Promise.race(file).then(() => {
// 放到zip统一下载
downloadZip(row.name, downloadList.value)
})
}
else {
ElMessage.error('未上传下载文件')
}
},
},
])
标题:利用JSzip进行多类型文件下载(pdf、图片类型)
作者:mcwu
地址:http://mcongblog.com/articles/2024/04/09/1712631477922.html
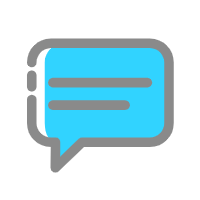
0 评论