ECMAScript2023(ES14)新特性简介
前段时间闲来无事,在翻看MDN文档时无意中发现Array中多了一些陌生的API,后来通过百度发现这些API都是在2023 年 6 月 27 日发布的ES14版本中新增的数组方法,于是便根据MDN一步一步研究了一下,发现有些API确实是比原来的API更加的好用,下面就一起来看一下吧~~~
Array.prototype.toSorted()
MDN对于这个API的描述是:
sort()
方法的复制方法版本。它返回一个新数组,其元素按升序排列。
toSorted()
方法有以下几种常见的用法:// 1. 不传入函数 toSorted() // 2. 传入箭头函数 toSorted((a,b) => { /**……**/ }) // 3. 传入比较函数 toSorted(compareFn) // 4. 内联比较函数 toSorted(function compareFn(a, b) { /**……**/ }) // toSorted数组排序 let arr = [3,4,2,10,1,6,9,5,8,7] const sort = arr.toSorted((a,b) => b-a) // [10, 9, 8, 7, 6, 5, 4, 3, 2, 1] const sort2 = arr.sort((a,b) => a-b) // [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] // 以上两个排序看上去没有区别,那么就往下看 /** * arr未打开时显示的是[10, 9, 8, 7, 6, 5, 4, 3, 2, 1],打开后显示的是[1, 10, 2, 3, 4, 5, 6, 7, 8, 9] 不理解的请看下边的图 * 原因:当调用arr.sort()后,因为没有传入参数, * 它默认会按照Unicode字符的顺序来排序, * 另外因为浏览器本身具备懒加载的功能, * 在打开数组时才会去从新计算数组中的数据(按照Unicode字符排序) * 打开时时代码都执行完了,所以受到了arr.sort()的影响,它会从[10, 9, 8, 7, 6, 5, 4, 3, 2, 1]变成[1, 10, 2, 3, 4, 5, 6, 7, 8, 9] * 所以才会导致未打开时显示的是[10, 9, 8, 7, 6, 5, 4, 3, 2, 1],打开后显示的是[1, 10, 2, 3, 4, 5, 6, 7, 8, 9] * * sort()和toSorted()的区别: * sort()会改变原数组,toSorted()不会改变原数组, * 本质上就是sort()和原数组指向的堆内存地址是一致的 * 而toSorted()和原数组指向的堆内存地址不一致 * 但如果受到了sort()的影响,它也会根据sort()按照Unicode字符排序, * 但堆内存地址其实还是不同,类似于深拷贝和浅拷贝 * **/ arr = [10, 9, 8, 7, 6, 5, 4, 3, 2, 1] const isTrue = arr === arr.sort() // true const isTrue2 = arr === arr.toSorted() // false console.log('重新赋值后的',arr,arr.toSorted()) // [1, 10, 2, 3, 4, 5, 6, 7, 8, 9] (10) [1, 10, 2, 3, 4, 5, 6, 7, 8, 9] console.log(sort,sort2,isTrue,isTrue2)
需要注意的是,toSorted() 也可以应用于对象数组。这种情况下,需要提供一个使用对象上的数据的比较函数,因为对象没有自然的排序方式。// 比较对象 const objects = [ { name: "Harry Potter", age: 30 }, { name: "Neville Longbottom", age: 25 }, { name: "Hermione Granger", age: 40 }, { name: "Ron Weasley", age: 20 }]; const sortedObjects = objects.toSorted((a, b) => a.name.localeCompare(b.name) console.log(sortedObjects); // [{ name: "Harry Potter", age: 30 },{ name: "Hermione Granger", age: 40 }, { name: "Neville Longbottom", age: 25 },{ name: "Ron Weasley", age: 20 }]
Array.prototype.with()
MDN对于这个API的描述为:
with()
方法是使用方括号表示法修改指定索引值的复制方法版本。它会返回一个新数组,其指定索引处的值会被新值替换。其意思可以理解为如果想要根据下表修改数组内某个值,那么就看以用with()
,其语法也很简单// index 要修改的数组索引(从 0 开始)value 要分配给指定索引的任何值。 array.with(index, value) // with()根据下标修改内容 const arr2 = ['I','Love','You'] const With = arr2.with(1,"don't love") console.log(With,string) // ['I', 'don't love', 'You'] ['I', 'Love', 'You']
Array.prototype.toReversed()
MDN对于这个API的描述为:
toReversed()
方法是reverse()
方法对应的复制版本。它返回一个元素顺序相反的新数组。简单来说其实和reverse()
没有任何区别const arr2 = ['I','Love','You'] const With = arr2.with(1,"don't love") console.log(arr2.toReversed(),With.toReversed()) // ['You', 'Love', 'I'] ['You', "don't love", 'I']
Array.prototype.findLast
MDN对于这个API的描述为:
findLast()
方法反向迭代数组,并返回满足提供的测试函数的第一个元素的值。如果没有找到对应元素,则返回undefined
。// 通过下边代码发现,它是从数组最后开始查找并且返回这个值,如果没有就会显示为undefined const array1 = [5, 12, 50, 130, 44]; const array2 = [5, 12, 50, 130, 3]; const found = array1.findLast(item=> item % 2 === 0 ); const found2 = array1.findLast(item=> item % 2 !== 0 ); const found3 = array1.findLast(item=> item === 100 ); console.log(found,found2,found2); // 44 3 undefined
Array.prototype.findLastIndex
MDN对于这个API的描述为:
findLastIndex()
方法反向迭代数组,并返回满足所提供的测试函数的第一个元素的索引。若没有找到对应元素,则返回 -1。另请参见findLast()
方法,该方法返回最后一个满足测试函数的元素的值(而不是它的索引)。findLastIndex()
的工作方式与 findLast() 相同,只是它返回匹配元素的索引而不是元素本身。// findLastIndex() 的工作方式与 findLast() 相同,只是它返回匹配元素的索引而不是元素本身。 const array1 = [5, 12, 50, 130, 44]; const array2 = [5, 12, 50, 130, 3]; const found = array1.findLast(item=> item % 2 === 0 ); const found2 = array1.findLast(item=> item % 2 !== 0 ); const found3 = array1.findLast(item=> item === 100 ); console.log(found,found2,found2); // 4 0 -1
Array.prototype.toSpliced()
MDN对于这个API的描述为:
toSpliced()
方法是splice()
方法的复制版本。它返回一个新数组,并在给定的索引处删除和/或替换了一些元素。// 上面新增的所有方法也适用于 TypedArray,但 toSpliced() 这个新的数组方法只存在于 Array 中。 // toSpliced() 方法是 splice() 的复制版本,splice()是js中数组操作常用的方法; toSpliced() 方法会创建一个新数组,而不是修改原始数组。 const arr = ["red", "orange", "yellow", "green", "blue", "purple"]; const newArr = arr.toSpliced(2, 1, "pink", "cyan"); console.log(newArr); // ["red", "orange", "pink", "cyan", "green", "blue", "purple"] console.log(newArr[3]); // 'cyan' console.log(arr[3]);// 'green'
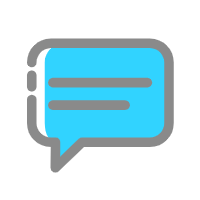