CSS让父盒子里的内容水平、垂直居中的写法
第一种:使用margin的方法
第一种方法是使用margin来使子盒子居中,但是这种方法会有一定的缺陷:需要给父盒子一个 overflow: hidden属性来清除塌陷,而且还需要精确计算上下的边距 如果父盒子改变大小,则还需重新计算大小
<style>
/*
第一种
使用margin来使父盒子里边的内容居中
缺点:需要给父盒子一个 overflow: hidden属性来清除塌陷,且还需要精确计算上下的边距 如果盒子改变大小,则还需重新计算大小
*/
.father {
width: 500px;
height: 500px;
background-color: pink;
overflow: hidden;
}
.son {
width: 200px;
height: 200px;
margin: 150px auto;
background-color: purple;
}
</style>
<body>
<div class="father">
<div class="son"></div>
</div>
</body>
第二种:使用定位加margin的方法
第二种方法是使用定位加外边距的方法来使子盒子居中,但是这种方法也会有一定的缺陷:如果盒子大小需要更改 同时需要更改外边距来让子盒子从新居中
<style>
.father {
width: 500px;
height: 500px;
background-color: pink;
position: relative;
}
/*
第二种
通过定位父盒子上和左各50% 然后再用外边距让盒子往上走自己宽高的一半
缺点:如果盒子大小需要更改 同时需要更改外边距来让子盒子从新居中
*/
.son {
width: 200px;
height: 200px;
position: absolute;
top: 50%;
left: 50%;
margin-top: -100px;
margin-left: -100px;
background-color: purple;
}
</style>
<body>
<div class="father">
<div class="son"></div>
</div>
</body>
第三种:使用定位加margin的方法(二)
第三种方法也是使用定位加外边距的方法来使子盒子居中,这样虽然不会出现两个盒子更改了宽高 而不居中问题 但需要将子盒子中的上下左右的距离全部设置一遍,比较麻烦
<style>
.father {
width: 500px;
height: 500px;
background-color: pink;
position: relative;
}
/*
第三种
通过对盒子定位 让其在父盒子中上下左右距离都为0 再通过margin=0的方法让其实现居中
这样不会出现子盒子更改了宽高 而不居中问题 但需要将上下左右的距离全部设置一遍,比较麻烦
*/
.son {
width: 200px;
height: 200px;
position: absolute;
top: 0;
bottom: 0;
left: 0;
right: 0;
margin: auto;
background-color: purple;
}
</style>
<body>
<div class="father">
<div class="son"></div>
</div>
</body>
第四种:通过定位加位移的方法
第四种方法是使用定位加位移的方法来使子盒子居中,这样既不会出现更改盒子宽高之后就不会居中的问题,而且相对于第三种方法来说代码量也少
<style>
.father {
width: 500px;
height: 500px;
background-color: pink;
position: relative;
}
/*
第四种
跟第上边第一种相似,也是通过对其定位的父盒子上和左各50%
然后使用transform属性来沿X轴与Y轴平移自己本身宽高的一半来设置
这样既不会出现更改盒子宽高之后就不会居中的问题,而且相对于第三种方法来说代码量也少
*/
.son {
width: 200px;
height: 200px;
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
background-color: purple;
}
</style>
<body>
<div class="father">
<div class="son"></div>
</div>
</body>
第五种:通过flex实现水平垂直居中
沿主轴和侧轴全部居中
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
* {
padding: 0;
margin: 0;
}
.father {
width: 300px;
height: 300px;
background-color: pink;
display: flex;
/* 主轴对齐水平 */
justify-content: center;
/* justify-content: space-around; */
/* justify-content: space-evenly; */
/* 侧轴居中对齐 */
align-items: center;
}
.son {
width: 100px;
height: 100px;
background-color: purple;
}
</style>
</head>
<body>
<div class="father">
<div class="son"></div>
</div>
</body>
</html>
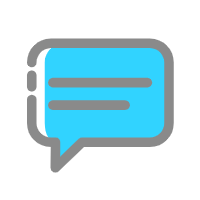
0 评论